We all know the feeling. You’re following a YouTube tutorial and coding along. Your code works as expected.
Then, at one point in the tutorial, you notice that you’re getting a different result from the tutor. But you followed and wrote every line of code as they did! Where’s the error coming from?
You rewind the video and delete the latest code. You then rewrite the last part—same error.
You can feel some frustration building up inside. Luckily, the YouTube video has a Github repo that you can refer to.
You visit the repository URL and countercheck your code. This is when you see it—a missing semicolon! You feel happy, but a little stupid.
What you’ve done is called “debugging”—finding errors in your code.
In this article, we answer the question what is debugging, and look at some of the errors that you can find during the process.
We also talk about the different kinds of debugging, the steps you take when finding bugs (errors in your code), some techniques and tools you can use to help you debug, and how to avoid bugs and errors in the first place.
- What is debugging?
- Types of debugging
- The five steps of debugging
- Debugging techniques
- Debugging tools
- How to prevent bugs
- Wrap-up
All ready to immerse yourself in the world of debugging? Then let’s begin!
1. What is debugging?
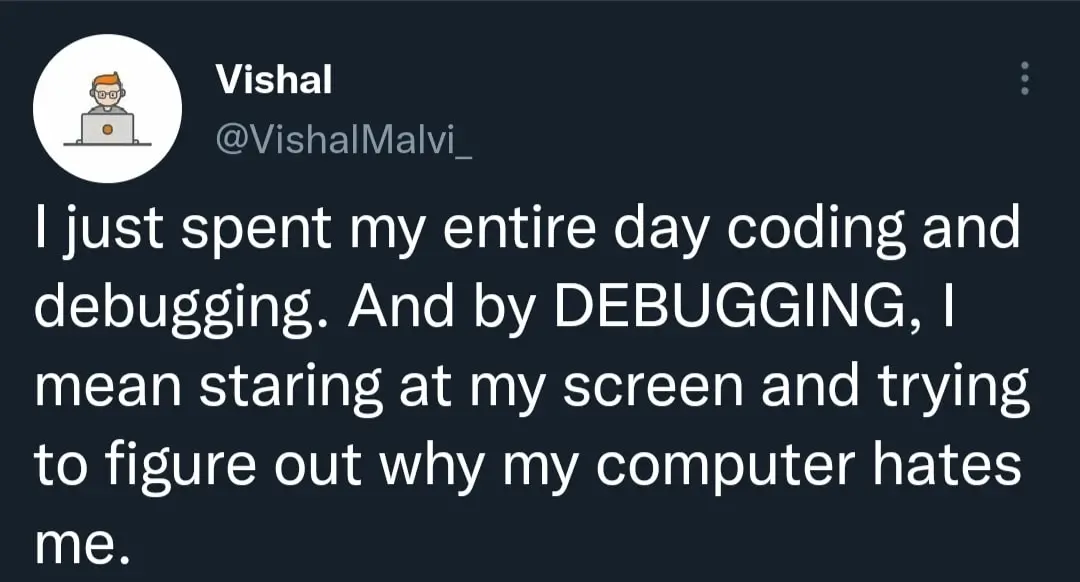
First of all, debugging doesn’t mean staring at your screen and wondering why your computer hates you.
Debugging is a process that involves identifying existing or even potential errors in a program. A program is a set of “instructions” that tell the computer what it needs to do.
For example, when you boot your computer and click on a browser like Google Chrome, it opens up.
A programmer wrote instructions for the computer to follow every time someone clicks on the browser icon.
Why is fixing errors in a program known as “debugging”? Let’s take a walk back in time.
A brief look at history
The term “bug” had been used before to describe mechanical malfunctions. The first person to use it to refer to computing problems was Admiral Grace Murray Hopper in the 1940s.
She worked in the navy, was a computer pioneer, and was working on the Mark II and Mark III computers.
Murray Hopper first used the term when she and her team found a moth inside their computer that was inhibiting its functioning. So, the term has a very literal origin!
Types of errors
There are various types of errors that can result in your program not doing what’s expected or failing to run at all. Let’s learn about them below:
Syntax errors
Syntax refers to the “rules” that define a programming language. Syntax errors, therefore, result from “breaking” programming language “rules.”
Syntax errors include typos or missing a semicolon.
Logical errors
A logical error occurs when your program doesn’t work the way it should. The program is able to execute, though.
Let’s look at an example.
for (var i = 1; i <= 10; i++) { if (i % 2 == 0) { console.log(i); } }
In the above for loop, we’re trying to generate all the even numbers below 10. The above code runs, giving the following even numbers: 2, 4, 6, 8, and 10.
However, there’s a problem with the results. Even numbers less than ten should include the number zero. Because we set our loop to begin at one instead of zero, we miss out on the first even number, zero.
Runtime errors
A runtime error occurs when you’re executing a program but execution stops. Sometimes, runtime errors can be caused by the environment in which you run your program.
There are many other types of errors that you’ll learn as you keep learning code. Better still, learn how to “catch” them in your code via concepts like “errors and exception handling.”
Phew! That was a lot of new concepts! Now that we’ve answered the question “What is debugging?” let’s learn about the various types.
2. Types of debugging
Debugging can be largely categorized into two types: reactive and proactive. Let’s dig deeper and learn a little more about them.
Reactive debugging
This is done after the bug has been identified. In the previous section, we looked at different types of errors.
All the types of errors we’ve come across so far are resolved via reactive debugging.
Proactive debugging
In proactive debugging, developers write code (as part of the program) that’s on the “lookout” for errors.
This “extra” code doesn’t affect the functionality of the rest of the program.
Proactive debugging is also referred to as preemptive debugging.
Proactive debugging is a part of both defensive and offensive programming, which are two other types of programming.
A word on remote debugging
It’s important to learn remote debugging as you become a more experienced developer. This is because the way in which we structure software changes a lot.
For example, we have more and more software running in the cloud. This means that we might not be able to debug some of the software locally on our computers.
Remote debugging is when you find bugs in an application that doesn’t run on your local computer. How cool is that?
We’ll delve more deeply into debugging types when we talk about techniques later on.
3. The five steps of debugging
In the quest to really understand what is debugging, there must be a way to make it more manageable and, who knows, maybe even pleasant.
Let’s discuss five simple steps to do this:
Step One: Gather information about the error
As a beginner programmer, you’re highly likely to make syntax errors. As a result, keeping an eye on these should be high on your priority list. Another thing you need to do is read error messages and try to understand them.
If you’re working with any data, for example, user data, check that too. You may have forgotten to perform data validation checks.
Step Two: Isolate the error
In this step, the goal is to try and identify the source of the error. You might need to “recreate” the error so as to know when it happens.
You could also comment out parts of the code and keep checking to see what section of your program could be causing the error.
You can also add print statements within your code (console.log in JavaScript). For example, if you’re able to print the output of a function, then it isn’t likely to be the one causing the error.
Keep doing this after every few lines of code. Eventually, you’ll see the section of your code that’s causing the error.
Step Three: Identify the error
Once you’ve narrowed down the section of the program that’s causing the error, you need to find the error itself. A great place to start is with a hypothesis.
For example, if you find that an error is coming from a field on your form, your hypothesis might be that you’re using the wrong data type.
You might be trying to add strings and integers, for instance.
Step Four: Determine how to fix the error
Once you have your hypothesis, it’s time to test it. For example, you might add data conversion logic to your program and run it to see if the error is gone.
If the error is still there, formulate another hypothesis and keep testing it. Keep doing this until the error is fixed.
Sometimes the solution to an error might not be obvious. You can visit sites like StackOverflow and find out whether anyone has experienced the same error and how they fixed it.
You might also ask a colleague for help or talk to an inanimate object and explain to it what you’re trying to do with your code (rubber duck debugging).
And now that we have ChatGPT, you can also ask it to help you find the error in your code and how to fix it.
Step Five: Apply and test
You can rerun the code to see if the error is fixed. Testing is a great way to ensure that you don’t end up creating more bugs when fixing the ones you’ve been focusing on!
Sometimes, you might not be able to figure out how to fix the error, despite your best efforts and even those of your colleagues.
In these situations, you’ll need to settle for a workaround that can keep the program running while you continue to find ways to completely get rid of the error.
An important thing that you might forget here is to document your solution. Yes, fixing the bug is a great achievement, and you feel elated. But documenting how you solved the error will help someone else in your team who might have encountered the error, or even your future self.
4. Debugging techniques
As a developer, debugging is part of your daily job. How often? Well, it could happen every eight minutes and go on for anywhere between a few minutes and 100 minutes.
With that in mind, what are a few things that you can do to make it faster, more efficient, and maybe even fun?
Active debugging
This is a technique that involves reducing the number of lines of code you’re going to actually debug.
One way of doing that that we’ve seen is to comment out pieces of your code until you narrow down to the section that’s causing the bug.
Adding print statements
You can add print statements after every few lines of code, or even after a line of code, to try and narrow down the section that’s causing the error.
Using a debugger
You don’t always have to find all the bugs yourself. You can enlist the help of a debugger (software that helps you find bugs).
Some IDEs (Integrated Development Environments) and text editors have built-in debuggers.
IDEs and text editors are just big words for where you write your code. Some IDEs and text editors include Atom, Visual Studio Code (VS Code), Android Studio, PyCharm, or Arduino IDE.
The IDE or text editor you use depends on the language you’re writing your program in or the platform you’re building for—web, Android, iOS, or even IoT.
VS Code, for example, has a built-in debugger to help you find bugs in your Node.js applications. It also works with JavaScript, TypeScript, and other languages that can be converted to JavaScript.
For other languages, you’ll need to install extensions from the VS Code Marketplace.
Using breakpoints
These are points in your code where execution stops—not because there’s a bug, but because you “paused” it.
It’s literally putting a “pause” on your code so that you can find bugs.
You can add breakpoints in your IDE, text editor, or even in your browser’s developer tools (you can access this on Chrome by pressing F12 on Windows and Linux, and Cmd + Opt + I on macOS).
Writing tests
All the techniques we have looked at so far can be categorized as “reactive debugging.” Writing tests is proactive debugging.
When writing tests, developers write a simple program that mimics what the larger program will do. This program is what’s referred to as a “test.”
They then run it (using a library like Mocha or Jasmine for JavaScript) to see any errors that may occur. The errors are then fixed until the code works.
Essentially, you’ll have created an “example” of the code you should write.
Developers should write tests even before they begin writing code. It’s standard practice.
At a beginner level, you’re going to mostly write unit tests.
Proactive debugging isn’t foolproof, which is why we still need reactive debugging methods.
5. Debugging tools
A critical part of exploring what is debugging is knowing which tools to carry in your arsenal.
A lot of the techniques that we learned involve the use of debugging tools. We’ve already come across some of the tools.
Note that these tools are highly dependent on the platform you’re working with, as well as the programming language you’re writing your program in.
Sometimes, the tool you choose to work with is a matter of preference, use case, or even experience.
Let’s delve into the tools.
Debuggers
Debuggers are basically tools that help you find bugs in your program. We’ve already come across debuggers. There are built-in debuggers like the one in VS Code.
Version control systems
You’ve probably used GitHub to store your code. If so, then you’ve come across Git, the version control system that makes it possible to keep track of your code changes.
Git can help you debug. Especially where you need to compare different versions of your code (something called “comparison debugging”).
You can also revert to previous versions of your code.
Testing frameworks
We mentioned that you could write tests when writing your code. The frameworks that can help you run these tests are known as “testing frameworks.”
They include Mocha and Jasmine in JavaScript or PyTest and Lettuce for Python.
Browser debugging tools
Most browsers have debug consoles. Chrome has Chrome Developer Tools, and Firefox has Firefox Developer Tools. On Windows and Linux, you can get to both by pressing F12, and on macOS, you need to press Cmd + Opt + I.
Rubber duck debugging
Unlike other tools on this list, rubber duck debugging isn’t software. It’s a way to find bugs by talking to a rubber duck.
You explain your code and what you’re trying to achieve to the rubber duck. As you do so, you’re highly likely to see where the bug is coming from.
The rubber duck can be a pet, any inanimate object, or another human (a colleague or really anyone).
AI debugging tools
We now have more options to help us debug, thanks to Artificial Intelligence (AI). As mentioned earlier, you can copy and paste your code into generative AI coding assistants like ChatGPT, and the tool will help you find the bug.
ChatGPT can even give suggestions on how to fix the bug.
Other AI coding assistants that can help you debug include GitHub Co-pilot and codeQL.
AI isn’t going to steal developer’s jobs: instead a lot of these AI tools, like SonarQube and CodeQL, can help with code analysis and identify vulnerabilities.
6. How to prevent bugs
The last part of really mastering your comprehension of what is debugging is knowing how to prevent bugs in the first place.
The truth is, there’ll never be a day when you write 100% bug-free code. This doesn’t mean that you should give up and just write “buggy code,” though.
Your goal should be to write code with as few bugs as possible. And when there are errors, they should be easier to find.
We’ve already discussed ways to prevent bugs. Let’s review them and learn a few more ways.
Writing tests
Writing tests helps you write significantly better code. With them, you’ll be able to take care of “edge cases,” for example. Edge cases are the other scenarios that your code needs to factor in.
For example, if you’re asking users to fill out a form, you need to add an extra step to ensure that the data entered is valid.
If you ask for an email address, then you need to add in checks containing the “@” symbol and a domain name like “.com.”
Writing tests will help you test out these scenarios even before you actually write the program.
Writing pseudo-code
Pseudo-code is what you’d want a user to do with your code. It’s written in human, not programming, language.
It assists you in breaking down your code into the most basic steps possible. This way, you’re able to clearly see what you’re going to write in your program.
For example, if you want your users to fill out a form, your pseudo-code might look like this:
1. Fill in the first field (first name).
If (name > 1)
Check whether they have two names and disable the next field.
elif (name is still > 1)
Allow them to fill in their first name and highlight the next field (the assumption is that they have two first names).
Else:
The next field should be highlighted.
1. Fill in the second field (last name).
If (name > 1)
Check whether they have two names and disable the next field.
Else:
The next field should be highlighted
As you can see, pseudo-code isn’t written in any particular programming language. However, it can help you break down your code step by step.
Writing simple code
As a rule of thumb, the simpler your code, the better. It’s easy to find a bug when you have simple code.
A good example is functions. A function should always do one thing. This way, if you’re trying to find errors in your code and you comment out a function, it’s easier to track it down.
Using modules
Modules are files with code that you can use in other files. Keeping your code modular is one way to keep it simple.
Instead of having 3000 lines of code, you can have several files with different aspects of the code. If you need to use code from another module, you can import it into your current module.
7. Wrap-up
In this article, we’ve thoroughly answered any questions about what is debugging. We explored its origins, the three main types of errors you’re likely to find, and even the two main types of debugging.
We learned steps that can make debugging a more logical process and hopefully a more pleasant experience, as well as techniques and tools that can help you find those bugs and smash them faster and more efficiently.
Ultimately, we went back to the root of the problem and asked ourselves how we could prevent errors in the first place.
We hope that you’re now ready to make finding bugs fun and, better yet, write code with significantly fewer errors.
If you’d like to get started coding from scratch, then try out this free 5-day short course, where you’ll learn to build your first website with HTML/CSS and JavaScript.
Want to read more about the world of web development? Check out these articles: